Telegram is a cloud based messaging application which provides an excellent set of APIs to allow developers to automate on top of the platform. It is increasingly being used to automate various notifications and messages. It has become a platform of choice to create bots which interact with users and groups.
Telethon is an asyncio Python 3 library for interacting with telegram API. It is one of the very exhaustive libraries which allows users to interact with telegram API as a user or as a bot.
Recently I have written some AWS Lambda functions to automate certain personal notifications. I could have run the code as a container on one of my VPSs or on Hulu or other platforms, but I took this exercise as an opportunity to learn more about serverless and functions. Also, my kind of load is something which can easyly fall under the Lambda free tier.
In this post we will look into the process of how to start with the development and write some basic python applications.
Registering As a Telegram Developer
Following steps can be followed to obtain the API ID for telegram –
- Sign up for Telegram using any application
- Login to the https://my.telegram.org/ website using the same mobile number. Telegram will send you a confirmation code on Telegram application. After entering the confirmation code, you will be seeing the following screen –
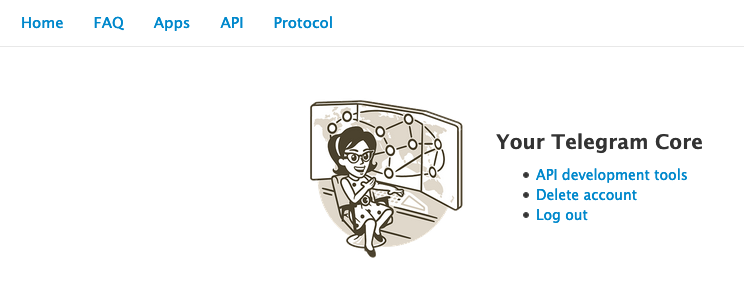
- In the above screen select the API Development Tools and complete the form. This page will provide some basic information in addition to api_id and api_hash.
Setting up Telethon Development Environment
I assume that the reader is familiar with basic python and knows how to set up a virtual environment, so rather than explaining, I would more focus on quick code to get the development environment up and running.
$ mkdir telethon-dev && cd telethon-dev
$ python3 -m venv venv-telethon
$ source venv-telethon/bin/activate
(venv-telethon) $ pip install --upgrade pip
(venv-telethon) $ pip install telethon
(venv-telethon) $ pip install python-dotenv
Obtaining The Telegram Session
I will be using .env
file for storing the api_id
and api_hash
so that the same can be used in the code which we will write. Replace NNNNN with your api_id
and XX with your api_hash
TELEGRAM_API_ID=NNNNN
TELEGRAM_API_HASH=XXXXXXXXXXXXXXXXXXXXXXXXXXXXXX
Next we will need to create a session to be used in our code. For full automation, it is needed that we store the session either as a file or as a string. Since the cloud environments destroy the ephimeral storage they provide, so I will get the session as a string. The following python code will help obtain the same.
#! /usr/bin/env python3
import os
from dotenv import load_dotenv
from telethon.sync import TelegramClient
from telethon.sessions import StringSession
load_dotenv()
with TelegramClient(StringSession(), os.getenv("TELEGRAM_API_ID"), os.getenv("TELEGRAM_API_HASH")) as client:
print(client.session.save())
When this code is executed, it will prompt for your phone number. Here you would need to print the phone number with the country code. In the next step, an authorization code will be received in the telegram application which would need to be entered in the application prompt. Once the authorization code is typed correctly, the session will be printed as a string value on standard output. You would need to save the same.
(venv-telethon) $ ./get_string_session.py
Please enter your phone (or bot token): +91xxxxxxxxxx
Please enter the code you received: zzzzz
Signed in successfully as KKKKKK KKKKKKK
9vznqQDuX2q34Fyir634qgDysl4gZ4Fhu82eZ9yHs35rKyXf9vznqQDuX2q34Fyir634qgDyslLov-S0t7KpTK6q6EdEnla7cqGD26N5uHg9rFtg83J8t2l5TlStCsuhWjdzbb29MFFSU5-l4gZ4Fhu9vznqQDuX2q34Fyir634qgDysl9vznqQDuX2q34Fyir634qgDy_x7Sr9lFgZsH99aOD35nSqw3RzBmm51EUIeKhG4hNeHuF1nwzttuBGQqqqfao8sTB5_purgT-hAd2prYJDBcavzH8igqk5KDCTsZVLVFIV32a9Odfvzg2MlnGRud64-S0t7KpTK6q6EdEnla7cqGD26N5uHg9rFtg83J8t2l5TlStCsuhWjdzbb29MFFSU5=
I normally put the string session along with the API ID and Hash in the .env
file. All these three values would need to be protected and should never be shared with a third party.
For the next code, I will assume that you have used a variable TELEGRAM_STRING_SESSION. So the final .env file will look like below –
TELEGRAM_API_ID=NNNNN
TELEGRAM_API_HASH=XXXXXXXXXXXXXXXXXXXXXXXXXXXXXX
TELEGRAM_STRING_SESSION=YYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYYY
Sending a Message to A Contact
Now we have the ground work done, so we will write a simple python application to send a message to a contact. The important point to note here is that the recipient must be in your telegram contacts.
#! /usr/bin/env python3
import os
from telethon.sync import TelegramClient
from telethon.sessions import StringSession
from dotenv import load_dotenv
load_dotenv()
try:
client = TelegramClient(StringSession(os.getenv("STRING_TOKEN")), os.getenv("API_ID"), os.getenv("API_HASH"))
client.start()
except Exception as e:
print(f"Exception while starting the client - {e}")
else:
print("Client started")
async def main():
try:
# Replace the xxxxx in the following line with the full international mobile number of the contact
# In place of mobile number you can use the telegram user id of the contact if you know
ret_value = await client.send_message("xxxxxxxxxxx", 'Hi')
except Exception as e:
print(f"Exception while sending the message - {e}")
else:
print(f"Message sent. Return Value {ret_value}")
with client:
client.loop.run_until_complete(main())
Next Steps
The telethon API is quite versatile, a detailed API documentation can be find at https://tl.telethon.dev/. Hope this post will help the reader quickly start off with the telegram messaging with telethon module.
Hello, very interesting post. How do you actually write the telegram event handler to be a aws lambda function? Thank you in advance